LLVM for Beginners: Getting Started with LLVM IR
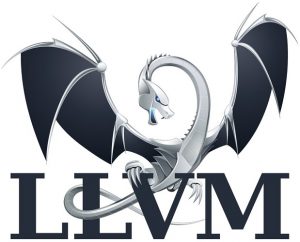
LLVM, standing for Low Level Virtual Machine, is more than a technology; it’s a foundation for building compilers and related tools. It’s a collection of modular and reusable compiler and toolchain technologies used widely in various programming languages.
In the modern development landscape, LLVM plays a pivotal role. It’s used for a variety of purposes, from developing compilers for new programming languages to implementing advanced optimization techniques in existing ones. Its flexibility allows developers to create more efficient and portable software.
Understanding LLVM IR
Definition of LLVM IR
LLVM Intermediate Representation (IR) is a low-level programming language used as a bridge in the LLVM compiler framework. It’s designed to provide a universal, intermediate form that can be converted into machine code.
Key Features
LLVM IR is notable for being platform-independent, which allows developers to write code that can be compiled on multiple architectures. Its human-readable form resembles assembly language but with high-level language features.
Setting Up the Environment
Prerequisites
To work with LLVM IR, you need a basic understanding of compilers and programming languages. Familiarity with command-line interfaces is also beneficial.
Installation Guide
To install LLVM, use your system’s package manager. For instance, on Ubuntu, you can use:
sudo apt-get install llvm
This command installs the LLVM suite, which includes the tools you’ll need to compile and work with LLVM IR.
Your First LLVM IR Program
Basic Structure
An LLVM IR program is composed of modules, each containing a list of functions and global variables.
Hello World Example
Here’s a simple example of an LLVM IR program that prints “Hello, World”:
@.str = private unnamed_addr constant [13 x i8] c"Hello, World\0A\00"
declare i32 @puts(i8* nocapture) nounwind
define i32 @main() {
%1 = call i32 @puts(i8* getelementptr inbounds ([13 x i8], [13 x i8]* @.str, i64 0, i64 0))
ret i32 0
}
This code defines a string and uses the puts
function to print it.
LLVM IR Syntax and Operations
Basic Syntax
The syntax of LLVM IR is relatively straightforward. It uses a type-first format, where each instruction’s type is declared before its operands.
Common Operations
Here are some common operations in LLVM IR:
add
: Adds two integers.fadd
: Adds two floating-point numbers.sub
,fsub
: Subtraction operations.mul
,fmul
: Multiplication operations.
Debugging LLVM IR Code
Tools and Techniques
LLVM provides several tools for debugging, like llvm-dis
and llvm-dwarfdump
. You can use these to inspect and debug your LLVM IR code.
Common Issues and Solutions
Some common issues include syntax errors and type mismatches. LLVM tools typically provide descriptive error messages to help identify and resolve these issues.
Advanced Topics in LLVM IR
Optimization Techniques
LLVM offers various optimization passes that can be applied to LLVM IR code to improve performance. These include dead code elimination, loop unrolling, and more.
Cross-Platform Capabilities
One of LLVM’s most significant advantages is its ability to target multiple architectures. You can write LLVM IR code once and compile it for different platforms without any changes.
Integrating LLVM with Other Languages
C/C++
LLVM can be integrated with C/C++ using the Clang compiler, which compiles C/C++ code to LLVM IR. This allows developers to leverage LLVM’s optimizations in their C/C++ projects.
Other Languages
LLVM also supports other languages like Rust, Swift, and more. These languages use LLVM for their backend compilation process, benefiting from its optimization and cross-platform capabilities.
LLVM Ecosystem and Community
Open Source Contributions
LLVM is an open-source project with a large and active community. Developers can contribute to its codebase, helping to improve and extend its capabilities.
Finding Help and Resources
The LLVM community provides extensive documentation, mailing lists, and forums where developers can seek help and discuss LLVM-related topics.
LLVM IR Best Practices
Coding Standards
Following coding standards is crucial for maintainability and readability. LLVM has its own set of coding standards that developers are encouraged to follow.
Performance Tips
To write efficient LLVM IR code, you should understand how different LLVM IR instructions translate to machine code and how different optimizations can affect your code.
Future of LLVM
Upcoming Features
The LLVM project is continuously evolving, with new features and improvements being added regularly. Keeping an eye on the official LLVM project page or its GitHub repository is a good way to stay updated.
Industry Impact
LLVM is expected to continue its growth in the software industry, with more languages and tools adopting it for their compiler infrastructure.
Case Studies
Real-World Applications
LLVM has been used in various large-scale projects, including the development of programming languages like Rust and Swift. It’s also used in several commercial and open-source compilers.
Success Stories
Many companies have successfully integrated LLVM into their projects to improve performance and portability. These case studies serve as a testament to LLVM’s capabilities and flexibility.
Comparing LLVM with Other Tools
Advantages and Disadvantages
While LLVM offers several advantages like flexibility and performance optimization, it also has a steeper learning curve compared to some other tools.
Use Case Scenarios
LLVM is particularly useful in scenarios where performance optimization and cross-platform support are crucial, such as in system software and game development.
Learning Resources
Books and Online Courses
There are several books and online courses available for learning LLVM. “Getting Started with LLVM Core Libraries” by Bruno Cardoso Lopes and Rafael Auler is a great starting point.
Community Forums and Groups
The LLVM community forums and groups are invaluable resources for learning and discussing LLVM-related topics.
Conclusion
LLVM and its Intermediate Representation offer incredible opportunities for software optimization and cross-platform development. Whether you’re a beginner or an experienced developer, diving into LLVM can open up new avenues in your programming journey.
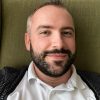
CO-FOUNDER & TECHNICAL LEAD
Experienced Senior Software Engineer with a demonstrated history of working in the telecommunications industry. Strong engineering professional skilled in Erlang, Elixir, Python, Java, C, Enterprise Software, VoIP, Linux.